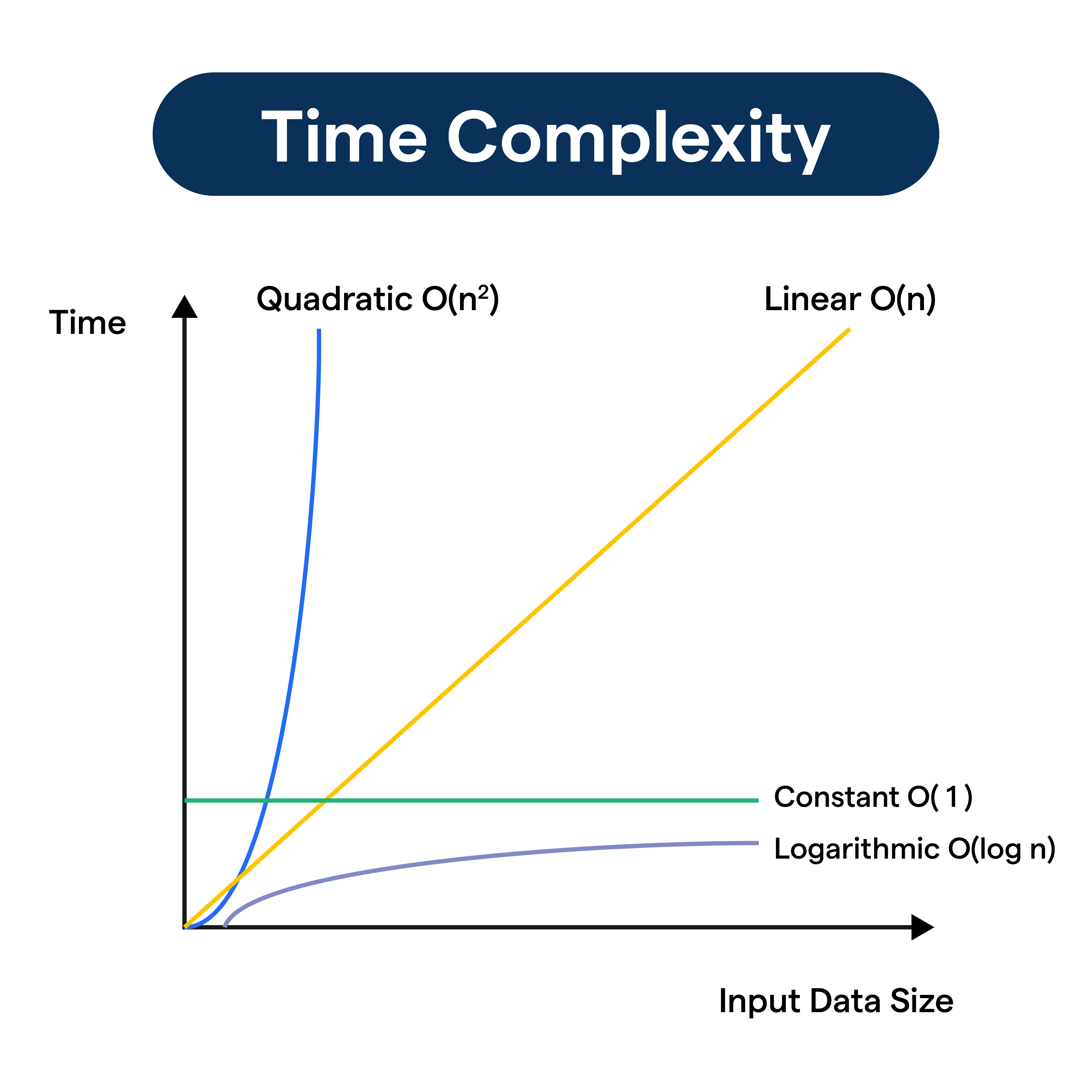
π Demystifying Time Complexity: A Guide for Developers
- Sravanthi
- Algorithms , Computer Science , Programming
- 02 Jan, 2025
Time complexity is a crucial concept in computer science, helping developers measure and optimize the efficiency of their algorithms. π§ β¨ This post explores what time complexity is, why it matters, and how you can apply it to write better code. Letβs dive in! π
π What is Time Complexity?
Time complexity refers to the amount of time an algorithm takes to run relative to the size of its input. Itβs usually expressed in Big-O Notation, which describes the upper bound of an algorithmβs runtime in the worst-case scenario. For example:
- ( O(1) ): Constant time
- ( O(n) ): Linear time
- ( O(n^2) ): Quadratic time
π Why is Time Complexity Important?
- Performance Matters: Efficient algorithms save time and resources.
- Scalability: Helps ensure your code works with large datasets.
- Optimization: Identifies bottlenecks in your program.
π§ Common Big-O Notations
1. Constant Time β ( O(1) )
The runtime does not depend on the input size. Example: Accessing an array element by index.
def get_first_element(arr):
return arr[0]
2. Linear Time β ( O(n) )
The runtime grows linearly with the input size. Example: Iterating through a list.
max_value = arr[0]
for num in arr:
if num > max_value:
max_value = num
return max_value
3. Quadratic Time β ( O(n^2) )
Occurs with nested loops. Example: Bubble sort.
for i in range(len(arr)):
for j in range(0, len(arr) - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
π§ How to Analyze Time Complexity
1.Identify the loops: Each loop increases complexity. 2.Break down operations: Combine complexities of independent sections. 3.Consider recursion depth: Recursive calls add to the runtime.
π Optimizing Algorithms
Tips: β’Use efficient data structures (e.g., dictionaries, heaps). β’Avoid unnecessary nested loops. β’Prefer algorithms with lower time complexity, like merge sort over bubble sort.
π Conclusion
Mastering time complexity helps developers write efficient, scalable, and optimized code. With a solid understanding of these concepts, youβll be better equipped to tackle real-world problems and build high-performance applications! π‘β¨
HashNode Reference